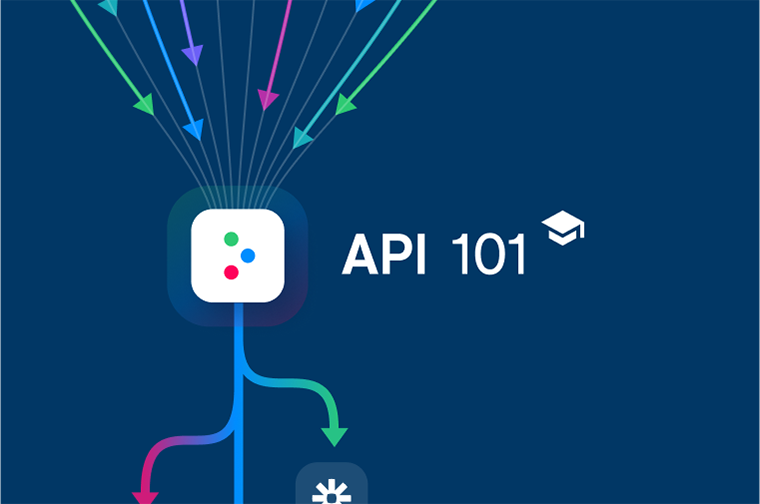
Talking to an API
The content in this guide won’t make you an expert on APIs (Application Programming Interfaces). But it will give you what you need to understand the basics of what’s happening.
Our focus will be on the structure of Web APIs. Once you understand that structure, you’ll be in a better place to understand the code in our other posts (or the ‘code’ your developer talks to you in!)
Let’s make a start. Just understand this first. A Web API is just a way of moving information between applications across the internet. That’s all it is. To use one, you just need to understand the structure of the call to the API. Let’s look at the structure step by step.
1. Authentication
Authentication is a big topic, but don’t worry and don’t get caught up in the detail. The authentication system is simply a way of proving who you are and getting a green light for access to the API. There are many ways of authenticating. Let’s just look at the ones you can use with the Datanow API. They broadly fall into two types: Datanow and OAuth.
Datanow authentication
If you’re starting out, Datanow authentication is the best place to begin. Very secure, but not that hard to use. Within Datanow authentication, there are three approaches.
1. Authenticate each time.
Like the name implies, each and every time you ask for data, you authenticate, that is you supply your user name and password. If they’re correct, you get given an Access Token (think of this as a looooooong pass-code). The Access Token is then immediately used when you request something else from the API.
2. Refresh Token.
This builds a little on the previous method. Instead of logging in each time, you re-use the Access Token you’re given. It sounds easy, but there’s one small snag - that the Access Token expires. To manage that, you get a second token called a Refresh Token. Think of the Refresh Token as the little wristband you get allowing you to leave a theme park and come back later in the day.
The benefit here is that you don’t need to keep supplying a user name and password - instead, Refresh Tokens are flying back and forth. Unfortunately, Refresh Tokens can expire too. You can avoid this by regularly refreshing them. If you don’t you’ll eventually become fully logged out. And you’ll need to start again….with your user name and password. Access Tokens tend to expire in hours, Refresh Tokens usually last days before they expire.
3. Permanent Key Token.
Finally, there’s a method that uses Tokens that never expire - ah, the convenience! It’s called API Key Authentication and is used by many Web services, including Datanow. The Permanent Key Token method is super easy to use. It involves storing the API key in your application, which is ok (compared with storing a user name and password in your application, which you should never do).
OAuth based authentication.
OAuth is the second authentication method supported by Datanow. At a beginner level however, you won’t want to deal with it. If you’re reading this and want to use it, get in touch with us by emailing info@datanow.co.nz.
2. Which method do you need?
Now that you’re proven who you are (authentication) it’s time to think about what you want to say to the API. The good news is that there are only two methods: GET and POST. And they are exactly as you would expect: for getting the latest values you use GET, and to send values you use POST.
3. What’s your endpoint?
An API is split into lots of endpoints. Think of an API as a large company - selecting the right endpoint is like finding the right division to take your request to.
Examples include:
- GET “the latest readings” =>
GET api.datanow.co.nz/timeseries/{TimeSeriesId}/data
- GET “latest shift notes” =>
GET api.datanow.co.nz/processes/{ProcessId}/shifts
Most APIs have a list of endpoints to help you find what you’re after. You can find the full list here Datanow API common specification.
4. What are the specific parameters?
Finally, we need to pay attention to the fine details - in the case of an API, it’s the parameters. Which sensor do you want the latest readings from, or what day did you want the shift note? This is done with ID values - replacing the {ProcessId} with a number. These numbers can be looked up using other endpoints.
The Datanow API also supports a fancy technology called OData. This lets you ask for data to be filtered or sorted in various ways. That way the API can do more work, and you can do less work in your code. An OData query has various extra bits on the end of the URL, like ?$top=1&$skip=2
.
Can you speak JSON?
We’ve just looked at making an initial ‘call’ to an API endpoint. Remember, the calls and responses supported by the API are described in an index. Datanow’s index can be found here Datanow API common specification.
But what about the content of the messages? The actual data being transferred by the Datanow API is in a format called JSON. Without going into the details of JSON, know that’s an easy to read, hierarcial format that nearly all Web APIs use. What this means it easy to share and use the information!
HTTP and status codes
There’s actually more information flowing than what we’ve described. That is, there’s more than just the authentication token, method, endpoint and parameters. The extra information is HTTP ‘packaging’. HTTP is the main ‘packaging system’ on the internet and all APIs use it.
You don’t need to understand the HTTP parts, but one useful piece is the status code. A status code tells us about the success, or failure of communications. It’s just a number and associated reason. A common list of status codes you might encounter when using and API is shown below.
- 200 - Success
- 400 - Request couldn’t be understood
- 401 - Unauthorised. You’re probably not authenticated
- 403 - Forbidden. You’re authenticated, but you don’t have permission to do whatever you tried to do.
An example
Here’s a common example, getting a list of downtime events from the Datanow API. To do this you would send…
GET https://api.datanow.co.nz/processes/101/events?$top=2
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpX...
If the request succeeds (in this case you would need to have authenticated before this call), you would get a response like.
[
{
"eventId": 29005947,
"start": "2020-05-20T09:45:14+12:00",
"end": null,
"note": null,
"quantity": 139.0,
"codeId": 1715,
"ratedSpeed": 20.0
},
{
"eventId": 29005674,
"start": "2020-05-20T08:15:08+12:00",
"end": "2020-05-20T09:45:14+12:00",
"note": "Stopping for product changeover",
"quantity": 0.0,
"codeId": 1709,
"ratedSpeed": 10.0
}
]
What we see here is just the JSON part of the response. That’s the bit we’re interested in. Yes, there is extra HTTP stuff flowing as well, but it’s of little interest. All we need to know that we succeeded (through a 200 status code) and the what the content of the response is.
This is the first time we’ve seen the actual JSON. As mentioned above it’s human readable. The downtime events (shown through field names and value pairs) are clear for all to see, and ready to use by another computer program.
What’s next
Now that we’re covered off some of the theory, it will be easier to follow along with our how-to guides which can be found on our website.